Warning: Root and flash your device at your own risk. I am not responsible when your device becomes unusable.
An Amazon Fire 7″ (2015 release) tablet recently came into my possession. The hardware is good considering the very low price. But I couldn’t stand FireOS, Amazon’s version of Android. It’s uglier. It has fewer features than stock Android. The Amazon Appstore has a worse selection of apps compared to Google Play. And every app on the tablet seems to try to sell you something.
I expelled Amazon from the tablet and installed a fully featured version of Android. It now runs like a dream.
You can follow along.
Required:
- Amazon Fire with FireOS 5.1.1 or less
- microSD card
Step 1: Enable ADB
- From your Fire, open Settings
- Tap Device Options
- Tap the device Serial Number seven times to reveal the Developer Options menu
- Tap Developer Options
- Set Enable ADB to on
Step 2: Root your tablet using SuperTool
- From your computer, download SuperTool from
http://forum.xda-developers.com/amazon-fire/development/amazon-fire-5th-gen-supertool-root-t3272695 - Run the SuperTool option: ADB and Fastboot Driver Install Plus Test
- Run the SuperTool option: Root your Amazon Fire 5th gen
- Run the SuperTool option: Install Google Play store
Step 3: Install FlashFire
- At the time for writing, FlashFire is in beta. You can signup for the beta using the following link. It may take 10 minutes after joining before you can install the app.
https://play.google.com/apps/testing/eu.chainfire.flash - Install [root] FlashFire by Chainfire from the Play Store
Step 4: Download Cyanogenmod 12.1
Download Cyanogenmod to your microSD card
http://forum.xda-developers.com/amazon-fire/orig-development/rom-cm-12-1-2015-11-15-t3249416
Step 5: Download SuperSU
Download Cyanogenmod to your microSD card
http://download.chainfire.eu/supersu-stable
Step 6: Download Google Apps
Download Google Apps to your microSD card
http://opengapps.org/
Platform: ARM
Android: 5.1
Variant: Nano
Step 7: Flash Cyanogenmod, SuperSU, and Google Apps
- Launch FlashFire.
- Click the red (+) button and choose Wipe.
Ensure System data, 3rd party apps and Dalvik cache are CHECKED. - Click the red (+) button and choose ‘Flash ZIP/OTA’.
Navigate to and choose the Cyanogenmod zip.
Ensure Auto-mount and Mount /system read/write are UNCHECKED. - Click the red (+) button and choose ‘Flash ZIP/OTA’.
Navigate to and choose the SuperSU zip.
Ensure Auto-mount is UNCHECKED.
Ensure Mount /system read/write is CHECKED. - Click the red (+) button and choose ‘Flash ZIP/OTA’.
Navigate to and choose the Google Apps zip.
Ensure Auto-mount is UNCHECKED.
Ensure Mount /system read/write is CHECKED. - Move “Wipe” to the Top of the order.
- Press the big FLASH button.
- Wait for reboot.
Done
At this point you should be running Cyanogenmod 12.1 with SuperSU for root and Google Apps.
Recovery
You can easily return to stock FireOS. You may need to do this if something goes wrong when installing Cyanogenmod. Or maybe you like FireOS better.
- Download FireOS 5.1.1
http://kindle-fire-updates.s3.amazonaws.com/8KNSnW7JKg3F3z46hiZnfi9TGa/update-kindle-global-37.5.4.1_user_541112720.bin - Download and run ADB Installer
http://forum.xda-developers.com/showthread.php?p=4891511 - Boot Fire into recovery: hold volume down button & power button at the same time. If it boots to fastboot mode, use the other volume button.
- Select Sideload
- Connect to PC via usb cable
- Open a command prompt with the path set to the folder where you downloaded FireOS
- Run this command to load the firmware:
adb sideload update-kindle-global-37.5.4.1_user_541112720.bin
- Once the recovery options come back, wipe data and cache.
- Select reboot
- For more help, see
http://forum.xda-developers.com/amazon-fire/general/unbrick-amazon-fire-7in-2015-5th-gen-t3285294
I released a new version (1.2.7) of GMinder today, with the following improvements:
The new Quick Add feature
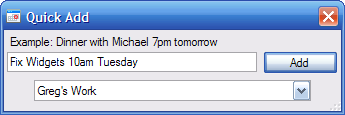
I recommend installing this new version, especially if a previous version had given you trouble.
Thank you for all your suggestions and feedback!